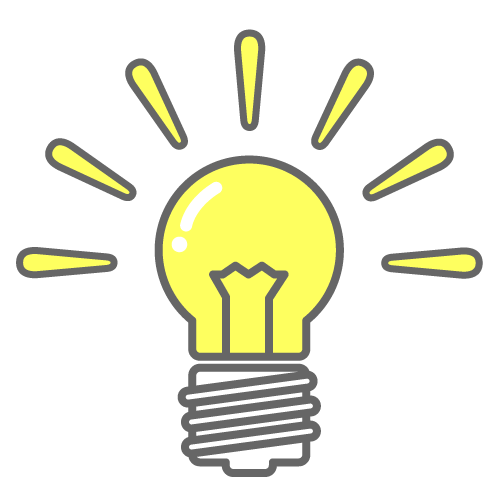
いきさつ
記事をtwitterにアップしてみたかった。
WordPress 4.1.7を使っているが、「Tweet old post」、「Tweetily」を使ったが正常に投稿できない。
「Buffer My Post」も使ってみたが、無料だと10回までしか投稿できなかった。
そのため、自作で対応した。
対応内容
script: python, bash
定期実行:cron
script
OAuth1Session、requests_oauthlib、MySQL-python、python-develが必要となる。
# easy_install OAuth1Session
# easy_install requests requests_oauthlib
# easy_install MySQL-python
# yum install python-devel
※mysql以外を使用している人は対象DB用のコネクタが必要
twit_up.py
#!/usr/bin/python # -*- coding: utf-8 -*- import MySQLdb import random import os from requests_oauthlib import OAuth1Session # # function to take out one at random # def random_choice_unique(lists, num=1): if not lists: return [] if len(lists) <= num: return lists copy_lists = lists[:] results = [] for i in range(num): t = random.choice(copy_lists) results.append(t) return results # # function to retrieve the article from db # def get_article(): connection = MySQLdb.connect(host="localhost", db="*****", user="*****", passwd="*****", charset="utf8") cursor = connection.cursor() # SQL cursor.execute("""select wpp.post_name , wpp.post_title , wpt.slug , CONCAT((SELECT `wp_options`.`option_value` FROM `wp_options` WHERE `wp_options`.`option_id` = 1), '/', wpt.slug, '/', wpp.post_name) AS 'url' from wp_posts wpp , wp_term_relationships wptr , wp_term_taxonomy wptt , wp_terms wpt where wpp.id = wptr.object_id and wptr.term_taxonomy_id = wptt.term_taxonomy_id and wpt.term_id = wptt.term_id and wpp.post_status in ('publish') and wpp.post_type = 'post' """) result = cursor.fetchall() r = random_choice_unique(result) param = {} for row in r: params = {"status": row[1]+" "+row[3]} cursor.close() connection.close() return params if __name__ == '__main__': CK = '**********************' # Consumer Key CS = '******************************************' # Consumer Secret AT = '********-*****************************************' # Access Token AS = '******************************************' # Accesss Token Secert # url for the tweet post url = "https://api.twitter.com/1.1/statuses/update.json" # tweet text params = get_article() # it will post in the POST methed with OAth authentication twitter = OAuth1Session(CK, CS, AT, AS) req = twitter.post(url, params = params) # we see the response if req.status_code == 200: print ("OK") else: print ("Error: %d" % req.status_code)
※consumer keyなどの情報は下記を参照した。
Twitterアプリケーションの作成
twit_up.sh
mysqlclientは別途インストールしたものを使用するため、作成。
私の環境では、postfixが古いmysqlclientを使っており、ld.so.confを書き換えられなかった。
そのため、プログラムごとに「LD_LIBRARY_PATH」を設定している。
システムで統一して同一のmysqlclientを使用する場合は、不要
#!/bin/bash export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:/usr/local/mysql/lib/ /root/script/twit_up.py
cron設定
4時間ごとに動作させる。
0 */4 * * * /root/script/twit_up.sh > /root/script/twit_up.log 2>&1
参考
ありがとうございました。
http://netaone.com/wp/tweet-old-post/
http://webnaut.jp/develop/633.html
http://qiita.com/ota42y/items/9918789e40f657494024
http://qiita.com/yubais/items/dd143fe608ccad8e9f85
http://d.hatena.ne.jp/s-n-k/20080512/1210611374
http://tt-house.com/2011/11/centos5-7-mysql5-5-pytho.html
http://linuxserver.jp/%E3%82%B5%E3%83%BC%E3%83%90%E6%A7%8B%E7%AF%89/db/mysql/%E5%A4%96%E9%83%A8%E6%8E%A5%E7%B6%9A%E8%A8%B1%E5%8F%AF%E8%A8%AD%E5%AE%9A
http://www.pythonweb.jp/tutorial/string/index6.html
http://website-planner.com/twitter%E3%82%A2%E3%83%97%E3%83%AA%E3%82%B1%E3%83%BC%E3%82%B7%E3%83%A7%E3%83%B3%E3%81%AE%E4%BD%9C%E6%88%90%EF%BC%88consumer-key%E3%80%81consumer-secret%E3%80%81access-token%E3%80%81access-token-secret/
以上